Introduction
RecyclerView in Android – Displaying lists in Android apps is common, whether it’s a list of contacts, news articles, or messages. In the past, developers used ListView
, but it had performance issues when handling large datasets. That’s why RecyclerView was introduced—it’s faster, more efficient, and highly customizable.
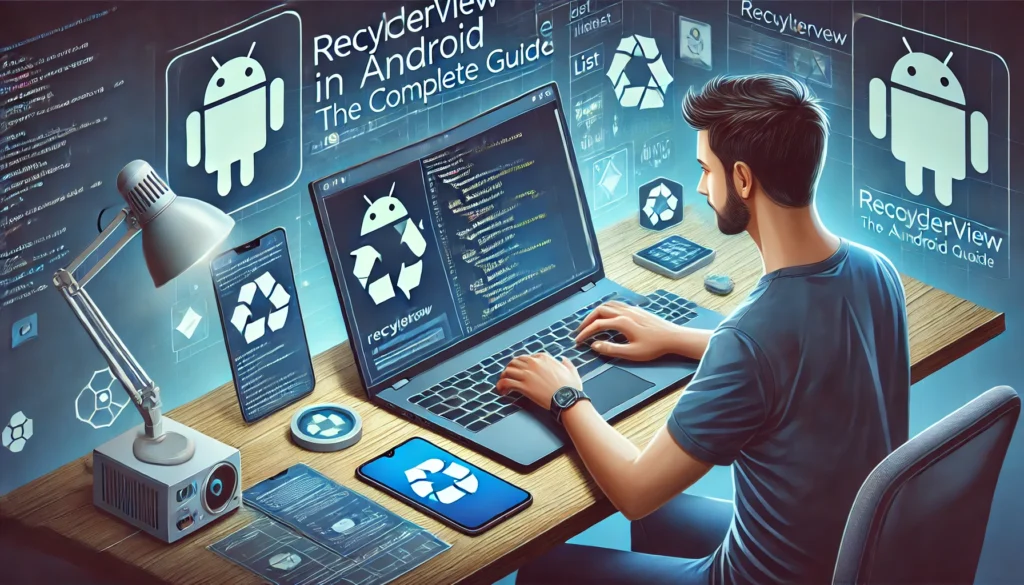
In this guide, we’ll explore what RecyclerView is, how it works, and how you can use it in your Android app.
What is RecyclerView?
RecyclerView is a flexible, optimized list-viewing component in Android. It efficiently handles large lists and grids by reusing views instead of creating new ones every time.
Why Use RecyclerView?
- Better Performance: Reuses item views instead of creating new ones.
- More Flexible Layouts: Supports linear, grid, and staggered layouts.
- Customizable Animations: Allows custom animations and decorations.
- Handles Large Data Efficiently: Works smoothly even with thousands of items.
How RecyclerView Works
RecyclerView has three main components:
- Adapter – Binds data to the UI.
- ViewHolder – Holds references to item views.
- LayoutManager – Defines how items are arranged (linear, grid, or staggered).
Step-by-Step Guide to Using RecyclerView
1. Add RecyclerView to Your Project
Make sure your project includes RecyclerView by adding the dependency to build.gradle
:
dependencies {
implementation 'androidx.recyclerview:recyclerview:1.2.1'
}
2. Add RecyclerView to Your Layout
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="16dp"/>
3. Create a Layout for List Items
Create an XML layout file (item_layout.xml
) for individual items in the list.
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:padding="10dp">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="18sp"/>
</LinearLayout>
4. Create a Data Model Class
public class Item {
private String text;
public Item(String text) {
this.text = text;
}
public String getText() {
return text;
}
}
5. Create a RecyclerView Adapter
public class MyAdapter extends RecyclerView.Adapter<MyAdapter.MyViewHolder> {
private List<Item> itemList;
public MyAdapter(List<Item> itemList) {
this.itemList = itemList;
}
@NonNull
@Override
public MyViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext())
.inflate(R.layout.item_layout, parent, false);
return new MyViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull MyViewHolder holder, int position) {
holder.textView.setText(itemList.get(position).getText());
}
@Override
public int getItemCount() {
return itemList.size();
}
public static class MyViewHolder extends RecyclerView.ViewHolder {
TextView textView;
public MyViewHolder(View itemView) {
super(itemView);
textView = itemView.findViewById(R.id.textView);
}
}
}
6. Set Up RecyclerView in Activity
RecyclerView recyclerView = findViewById(R.id.recyclerView);
recyclerView.setLayoutManager(new LinearLayoutManager(this));
List<Item> itemList = new ArrayList<>();
itemList.add(new Item("Item 1"));
itemList.add(new Item("Item 2"));
itemList.add(new Item("Item 3"));
MyAdapter adapter = new MyAdapter(itemList);
recyclerView.setAdapter(adapter);
Different Layout Managers in RecyclerView
RecyclerView supports multiple layout managers to control how items are displayed:
- LinearLayoutManager (Default) – Displays items in a vertical or horizontal list.
recyclerView.setLayoutManager(new LinearLayoutManager(this));
- GridLayoutManager – Displays items in a grid format.
recyclerView.setLayoutManager(new GridLayoutManager(this, 2)); // 2 columns
- StaggeredGridLayoutManager – Displays items in a staggered grid format.
recyclerView.setLayoutManager(new StaggeredGridLayoutManager(2, StaggeredGridLayoutManager.VERTICAL));
Adding Click Events to RecyclerView Items
To make items clickable, modify the onBindViewHolder
method:
@Override
public void onBindViewHolder(@NonNull MyViewHolder holder, int position) {
holder.textView.setText(itemList.get(position).getText());
holder.itemView.setOnClickListener(v -> {
Toast.makeText(v.getContext(), "Clicked: " + itemList.get(position).getText(), Toast.LENGTH_SHORT).show();
});
}
RecyclerView vs. ListView: Which One Should You Use?
Feature | RecyclerView | ListView |
---|---|---|
Performance | High | Low |
View Recycling | Yes | No |
Custom Animations | Yes | Limited |
Flexible Layouts | Yes | No |
RecyclerView is the better choice because it offers more flexibility, better performance, and modern features.
Final Thoughts
RecyclerView is a powerful tool for displaying lists efficiently in Android apps. It’s highly customizable, supports various layouts, and handles large datasets smoothly. Whether you’re building a chat app, product catalog, or social media feed, using RecyclerView ensures a better user experience.
Try implementing it in your next Android project and see the difference!
📌 Further Reading: RecyclerView Official Docs