Hi all, in this tutorial we will learn about the buttons in Flutter. Flutter provides various buttons to enhance user interaction. Buttons help users trigger actions, submit forms, and navigate through apps. In this guide, you’ll learn about different button types in Flutter, how to use them, and best practices for implementation.
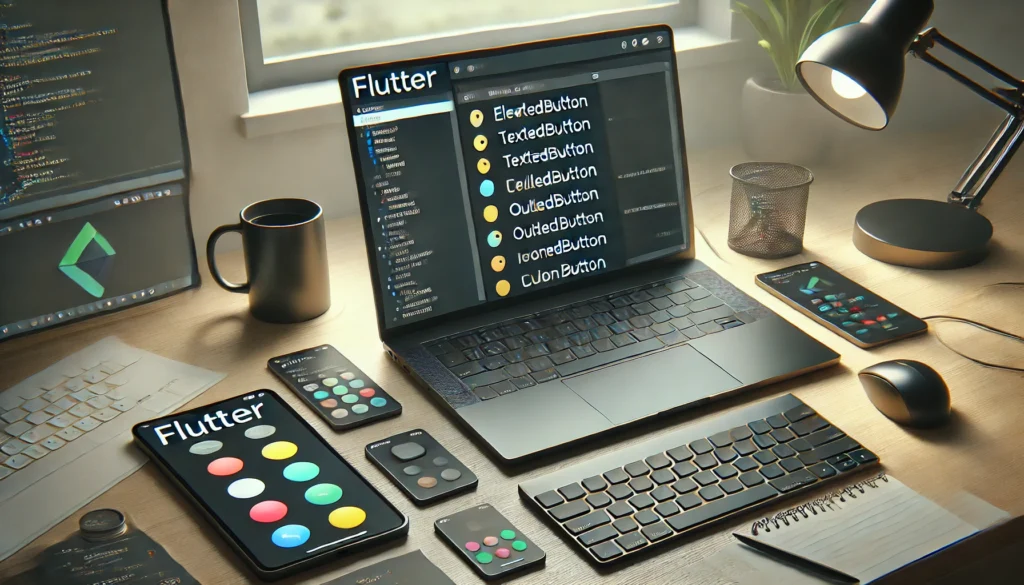
1. Types of Buttons in Flutter
Flutter offers several button widgets, each serving different purposes:
1.1 ElevatedButton
- A button with a shadow (elevation) for emphasis.
- Commonly used for primary actions.
ElevatedButton(
onPressed: () {
print('ElevatedButton clicked');
},
child: Text('Click Me'),
)
1.2 TextButton
- A simple text-based button without elevation.
- Suitable for secondary actions.
TextButton(
onPressed: () {
print('TextButton clicked');
},
child: Text('Click Here'),
)
1.3 OutlinedButton
- A button with an outline but no background.
- Ideal for actions that require less emphasis.
OutlinedButton(
onPressed: () {
print('OutlinedButton clicked');
},
child: Text('Tap Me'),
)
1.4 IconButton
- A button that displays an icon instead of text.
- Used for toolbar actions like back or menu buttons.
IconButton(
onPressed: () {
print('IconButton clicked');
},
icon: Icon(Icons.favorite),
)
1.5 FloatingActionButton (FAB)
- A circular button used for prominent actions.
- Often used for adding new content.
FloatingActionButton(
onPressed: () {
print('FAB clicked');
},
child: Icon(Icons.add),
)
2. Customizing Buttons
You can customize Flutter buttons using properties like:
- Style:
style: ElevatedButton.styleFrom(...)
- Color:
backgroundColor, foregroundColor
- Size:
minimumSize, padding
- Shape:
shape: RoundedRectangleBorder(...)
Example:
ElevatedButton(
style: ElevatedButton.styleFrom(
backgroundColor: Colors.blue,
foregroundColor: Colors.white,
padding: EdgeInsets.symmetric(horizontal: 20, vertical: 10),
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(10),
),
),
onPressed: () {
print('Custom button clicked');
},
child: Text('Custom Button'),
)
3. Button Click Events
To handle button clicks, use the onPressed
callback:
ElevatedButton(
onPressed: () {
print('Button clicked');
},
child: Text('Click Me'),
)
To disable a button, set onPressed: null
.
ElevatedButton(
onPressed: null,
child: Text('Disabled Button'),
)
4. Best Practices for Using Buttons in Flutter
- Use ElevatedButton for primary actions (e.g., Submit, Confirm).
- Use TextButton for less important actions (e.g., Cancel, Learn More).
- Use FloatingActionButton for key interactions (e.g., Adding a new item).
- Customize buttons to match the app’s theme for consistency.
- Ensure button accessibility (large tap areas and readable labels).
Conclusion
Flutter provides a variety of buttons for different use cases. By choosing the right button type and applying proper customization, you can improve the user experience in your app. Start implementing these buttons in your Flutter projects today!
Thanks you for reaching out us. You can comment for any query. Please share with your friend also and for more such tutorial you can look here.